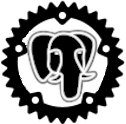
When Rust meets PostgreSQL
Elephantry is an open source database access framework in Rust dedicated to PostgreSQL.
let elephantry = elephantry::Pool::new(&database_url)?;
let results = elephantry
.execute("select name from department")?;
for result in &results {
let name: String = result.get("name");
println!("{name}");
}
Simple query
Connect to the database, execute a query a tell witch data you want and its type. Elephantry does the rest!
let elephantry = elephantry::Pool::new(database_url)?;
let results = elephantry
.query::<(String, String)>(include_str!("query.sql"), &[])?;
for result in results {
println!("{:result?}");
}
with ranking as (
select department.name as department,
employee.first_name || ' ' || employee.last_name as name,
rank() over (partition by department order by day_salary)
from employee
join department
on department.department_id = employee.department_id
order by department, rank
)
select row(department, name) from ranking
#[derive(Debug, elephantry::Entity)]
struct Employee {
id: i32,
name: String,
}
let employees = elephantry
.query::<Employee>("select id, name from employee", &[])?;
for employee in employees {
dbg!(employee);
}
Entity
Instead of retreive fields one by one, you can aggregate a row of result in a struct
.
Write data
You can easily retreive, create, update or delete entity with the model approach.
The cli helps you to generate entities from your database schema.
#[derive(elephantry::Entity)]
#[elephantry(model = "Model", structure = "Structure")]
struct Entity {
#[elephantry(pk)]
id: i32,
name: String,
}
elephantry.find_all::<Model>(Some("order by name"))?;
elephantry.insert_one::<Model>(entity)?;
elephantry.update_one::<Model>(pk!{id => entity.id}, entity)?;
elephantry.delete_one::<Model>(entity)?;
// config-support
let mut config = config::Config::new();
config.merge(config::Environment::with_prefix("DATABASE"))?;
let elephantry =
elephantry::Pool::from_config(&config.try_into()?)?;
// r2d2
let manager =
elephantry::r2d2::ConnectionManager::new(&database_url);
# rocket
[global.databases.elcaro]
url = "postgresql://localhost/elcaro"
Ecosystem
By enabling the corresponding feature (config-support
, r2d2
or rocket
), elephantry can easily integrate the rust ecosystem.
let r#async = elephantry.r#async();
r#async.execute("select * from department").await?;
let transaction = elephantry.transaction();
transaction.start()?;
// …
transaction.commit()?;